I recently came about the topic of an Entity-Component-System (ECS) - a different approach to handling GameObjects and Components - and I was intrigued. On closer inspection of this technique, I found that it was significalty faster than the traditional GameObject-Component approach.
Therefore, I decided to make my own implementation.
Therefore, I decided to make my own implementation.
The easiest part is the Entity. An Entity in an ECS is a simple ID that we generate, so that was a matter of generating a number and keeping track of it.

The Registry is the public interface that keeps track of all Entities and also adds Components to those Entities.
The next question is, how does an Entity know which Components it has?
We could store Entities and Components together in 1 big multi-map, but this would lead to poor performance, since maps don't have contigious storage.
We could store Entities and Components together in 1 big multi-map, but this would lead to poor performance, since maps don't have contigious storage.
My solution to this problem came in 2 parts:
1) Simulate a map, but have an array of pairs, such as std::vector<std::pair>. This would allow us to have a map-like structure, but with contigious array.
2) Instead of using strings to check for, and find Components (which a lot of implementations do), we can generate a compile-time hash from the Component name, and use that as a unique identifier for a specific component.
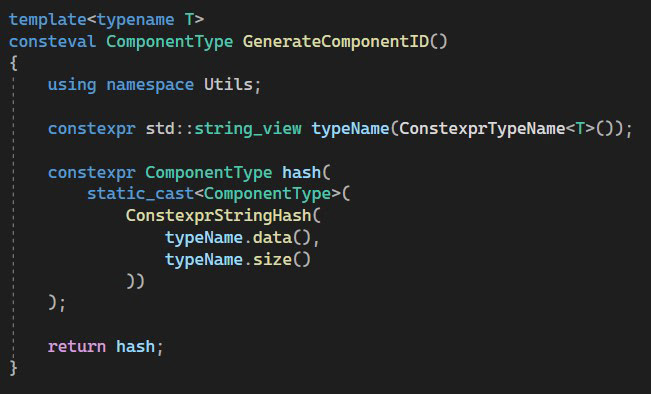
This function generates a unique Component ID at compile time.
This is done by getting the Component name - at compile time - and then hashing this name to generate a unique ID.
This is done by getting the Component name - at compile time - and then hashing this name to generate a unique ID.
Next, we can finally add the Component to our Entity.
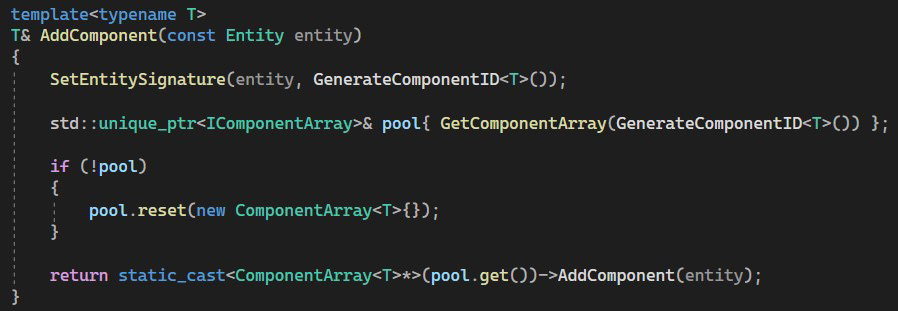
However, I have not discussed the most important part yet:
How fast is my ECS?
How fast is my ECS?
I benchmarked 2 seperate things:
1) Creation speed
2) Update speed
I performed these tests by:
1) Creating 1 million entities and adding 3 components to them.
2) Updating the formerly created 1 milllion entities' 3 components.
I ran these tests on 3 seperate design approaches:
The first one is my implementation of an ECS, the second one is a traditional GameObject - Component approach, and the final one is EntT, the ECS used by Minecraft (among others).
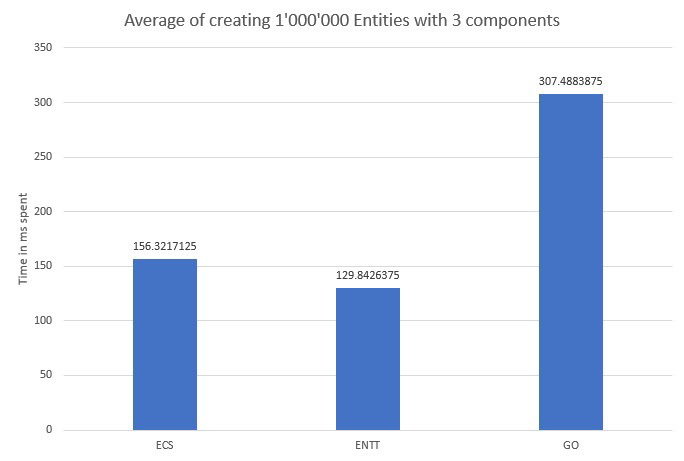
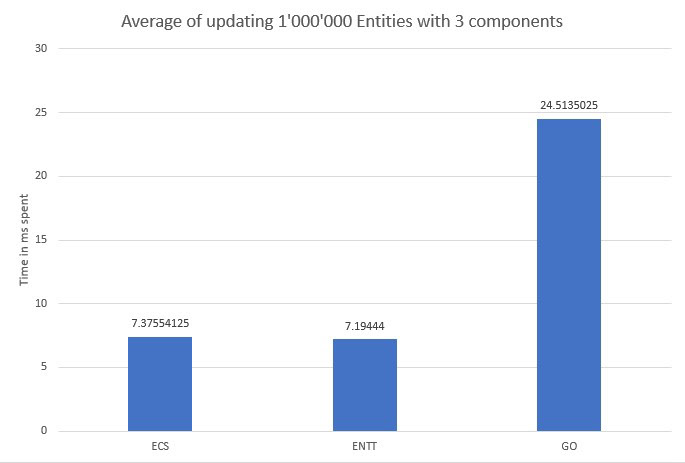
As you can see, both implementations of an ECS vastly outpace the traditional GameObject - Component approach, and EntT is slightly faster than my implementation.
I will not keep boring you with technical code snippets, so if you are interested in the entire code base, here is a link to the Github repository: https://github.com/Rhidian12/ECS